A Brief Introduction to Some of Common MATLAB Syntaxes and Usages
08/24/2019 Tags: MatlabPurpose
When designing on the algorithm implementation, the matlab script is one of the common programming languages to develope in the initial stage.
In this post, I recorded a few pieces of information in this post for avoiding to forget bit and pieces and will keep adding some informations.
Read data from text file
The fscanf is the common syntax to read data from text file.
Usage:
data = fscanf(filename, formatSpec)
Example:
filename = 'data.txt';
file = fopen(filename,'rt');
data = fscanf(file, '%x');
fclose(file)
Write data to text file
The fprint is the common syntax to write formatted data to file.
Usage:
fprintf(file_name, format, A1)
Example: Write 32 bits Data to Text File
filename = 'test.txt';
file = fopen(filename, 'w');
fprintf(file, '%08x ', 32767);
fclose(file);
Two’s complement
On the fixed-point design, we need to care about whether the fixed-point number is negative or not when doing two’s complement. Usually, we can use sign field to distinguish positive or negative.
Example:
% Check sign bit
sign_field = data >= (bit_len -1);
% Conversion (decimal)
if (sign_field)
data = - (2^bit_len - data);
Signed fixed-point numeric object
The matlab supports the constructures of signed and unsigned fixed-point numeric object, but unfortunately it does not have the overflowing protection, i.e The value can not more than 3 when the integral length is 3.
- sfi: With signed, two's-complement
- ufi: unsigned fixed-point numbers number
Usage:
sign_fixed = sfi(value, word length, fraction length)
Data Properties
bin — Stored integer value of a fi object in binary
data — Numerical real-world value of a fi object
dec — Stored integer value of a fi object in decimal
double — Real-world value of a fi object, stored as a MATLAB® double
hex — Stored integer value of a fi object in hexadecimal
int — Stored integer value of a fi object, stored in a built-in MATLAB integer data type. You can also use int8, int16, int32, int64, uint8, uint16, uint32, and uint64 to get the stored integer value of a fi object in these formats
oct — Stored integer value of a fi object in octal
Example:
b = sfi(3, 5, 2);
% binary representation
b.bin
% real-world value
b.data
% hexadecimal representation
b.hex
Read radio file
The audioread is the common syntax to write audio file, i.e., wav, mp3, au, flac, etc.
Usage:
[data,Fs] = audioread(filename)
, where data is the sampled data, and Fs is the samplerate for that data.
Example: Read a wav file
samples_frame = 640;
test_wav = sprintf('wav/test.wav');
[data, Fs] = audioread(test_wav);
numFrame = round(length(data) / (samples_frame));
time = (1:length(data))/Fs;
plot(time, data);
title('wave: s(n)');
Result:
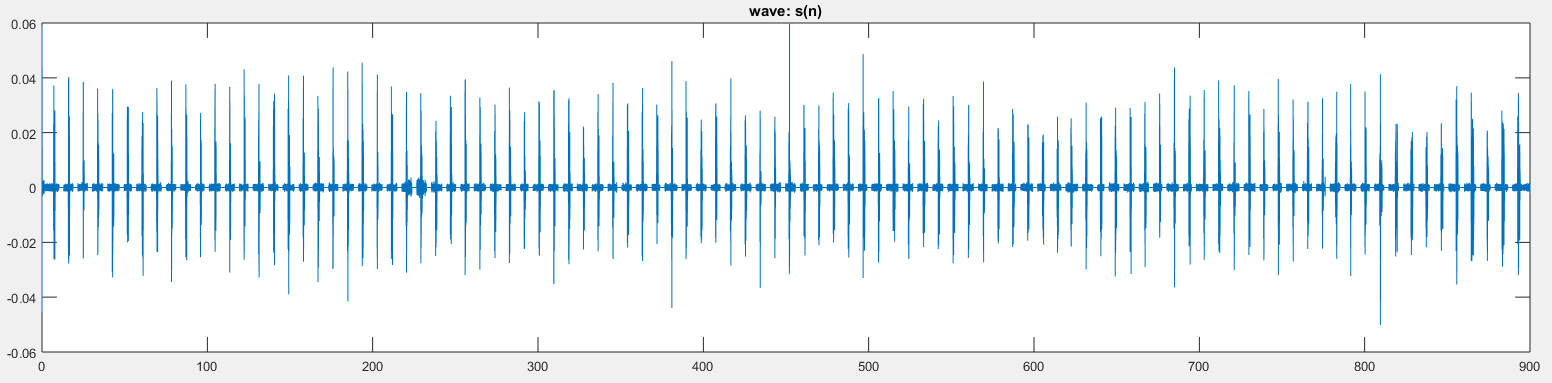
=========== To be continued…. ==========
Reference
[2] Write formatted data to file
[4] Construct signed fixed-point numeric object
[5] audioread
[6] Audio Signal Processing and Recognition (音訊處理與辨識)
Note
If you have any constructive criticism or advises, leave the comments below or feel free to email me qazqazqaz850@gmail.com. Hope this post will help! :)